In this tutorial, we will learn how to add logo to a home image that we have taken in OpenCV. We have two images, first one is Home and second one is Logo and we want to place logo on right side corner of the image.
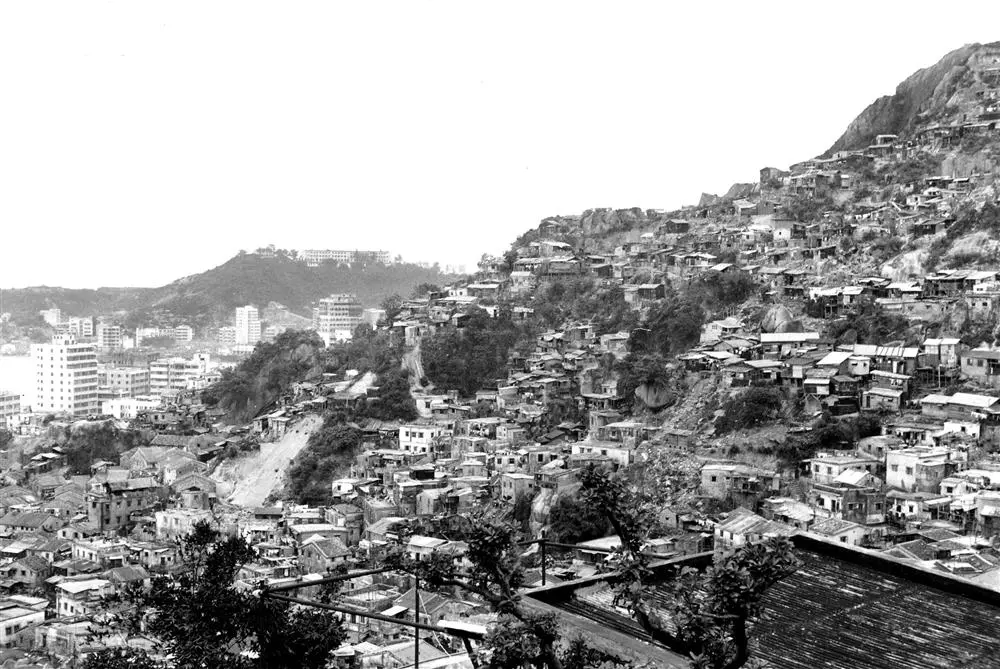
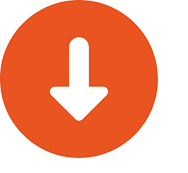
Step 1 – Use cv2.IMREAD_COLOR automatically deducts the channel of the image
Step 2- Convert Logo into Gray Scale image using cv2.COLOR_BGR2GRAY, so that we are working on same channel
Step 3- As we want to put logo on top left corner of home image, we create ROI
Step 4- Create a mask of the logo by binarization of image and applying that threshold value as mask to home image, also thresh specifies elements of the output array to be changed.
Step 5- When using bitwise_and() in opencv with python then white + anycolor = anycolor; black + anycolor = black. Also roi is added to src1 and scr2 as we don’t want two different images, but RULES defined in mask should be applied
Lets see the source code now-
import cv2
import matplotlib.pyplot as plt
image=cv2.imread("image3.jpg",cv2.IMREAD_COLOR)
logo=cv2.imread("image-3.png",cv2.IMREAD_COLOR)
logo = cv2.cvtColor(logo, cv2.COLOR_BGR2GRAY)
logo = cv2.resize(logo,(0,0),fx = 1.5,fy=1.5)
rows, cols = logo.shape
roi = image[0:rows, 0:cols]
retval, thresh= cv2.threshold(logo,127,255,cv2.THRESH_BINARY)
mask_Image= cv2.bitwise_and(roi, roi, mask = thresh)
image[0:rows, 0:cols] = mask_Image
cv2.imshow('mask_Image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
cv2.imwrite("mask_Image.jpg",image)
Output of the Image
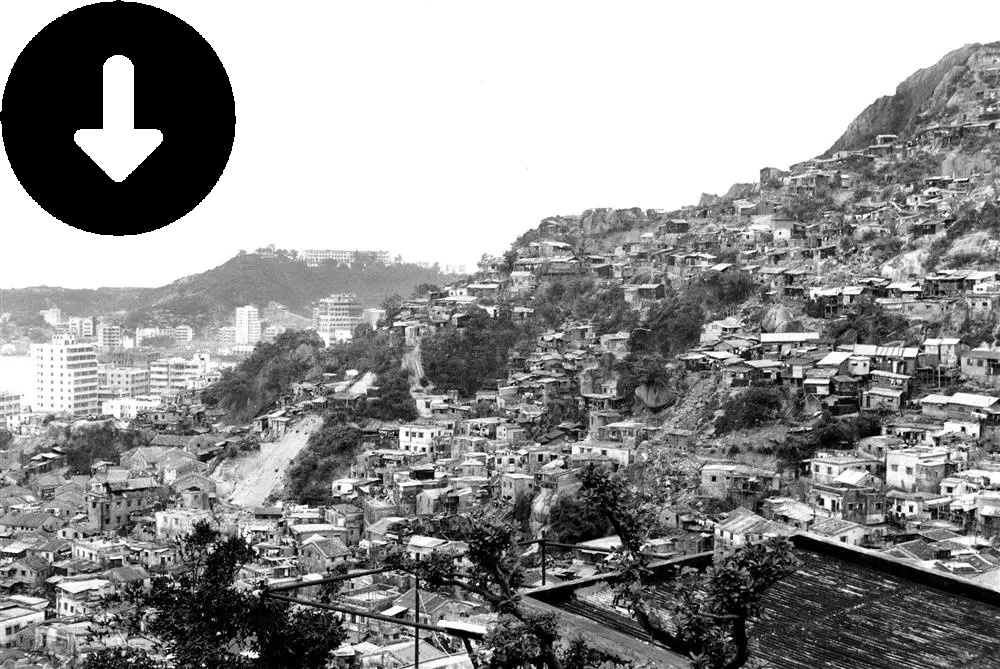